Learn how to create commands in few simple steps:
For creating commands in powerShell, I had installed templates for it, which I get from here: http://channel9.msdn.com/ShowPost.aspx?PostID=256835. There are VB.NET and C# ones.
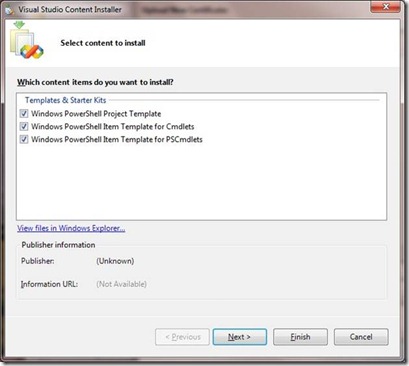
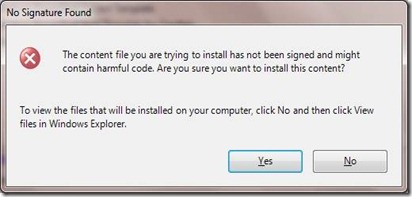
1) Now select a powerShell project as shown:
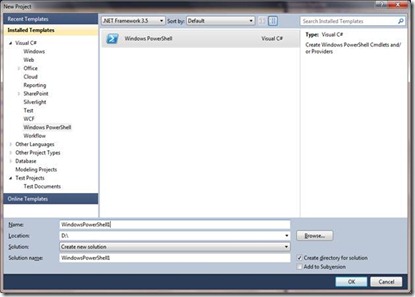
The project contains a PSSnapin file and a reference to the assembly System.Management.Automation
2) In the PSSnapIn.cs fill information as required.
[RunInstaller(true)]
public class WindowsPowerShell1SnapIn : PSSnapIn
{
public override string Name
{
get { return "MyFirstSnapIn"; }
}
public override string Vendor
{
get { return "Reeta"; }
}
public override string VendorResource
{
get { return "WindowsPowerShell1,"; }
}
public override string Description
{
get { return "Registers the CmdLets and Providers in this assembly"; }
}
public override string DescriptionResource
{
get { return "WindowsPowerShell1,Registers the CmdLets and Providers in this assembly"; }
}
}
3) Now add a new cmdlet class.
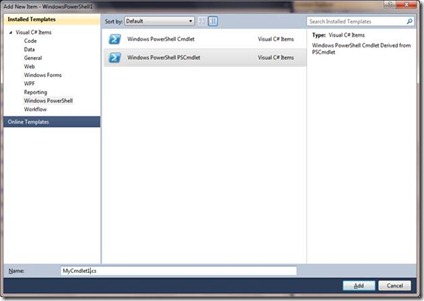
4) In this cmdlet we simply add a value to a parameter and output it.
[Cmdlet(VerbsCommon.Get, "Hello", SupportsShouldProcess = true)]
In this attribute “Get” is our verb and “Proc” is subject. Different verbs can be used. Read http://msdn.microsoft.com/en-us/library/ms714428(VS.85).aspx for more information.
5) To add parameter write :
[Parameter(Position = 0,
Mandatory = true,
ValueFromPipelineByPropertyName = true,
HelpMessage = "Enter a name")]
[ValidateNotNullOrEmpty]
public string Name
{
get;
set;
}
To validate a parameter we can add different attribute on a parameter. We can add help message for the parameter By “HelpMessage”. We can indicate that a parameter is mandatory by adding “Mandatory= true”. We can add position for a parameter.
6) Now add a code to write the parameter value in ProcessRecord(). When this command runs it execute code written in Processrecord().
WriteObject will write values on the commandlet.
7) For running the power shell we first need to install the dll.
On visual studio command prompt use - installUtil yourAssemblyPath.dll
8) Now add the SnapIn in the power shell using command:
Add-PSSnapin MyFirstSnapIn
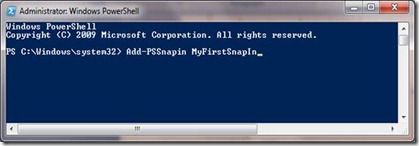
9) Now get the registered SnapIn list by command :
Get-PSSnapIn –registered
10) Now run the command using:
Get-Hello –Name Reeta
It will output – Hello Reeta
Since we had written position in the Parameter attribute we don’t need to specify –Name. if we write- Get-Hello Reeta, it gives same output.
If we don’t provide any parameter then it says that it is mandatory.
These are just simple steps for creating a command in powerShell. I get help from this blog http://blogs.msdn.com/b/daiken/archive/2007/02/07/creating-a-windows-powerShell-cmdlet-using-the-visual-studio-windows-powerShell-templates.aspx
For creating commands in powerShell, I had installed templates for it, which I get from here: http://channel9.msdn.com/ShowPost.aspx?PostID=256835. There are VB.NET and C# ones.
1) Now select a powerShell project as shown:
The project contains a PSSnapin file and a reference to the assembly System.Management.Automation
2) In the PSSnapIn.cs fill information as required.
[RunInstaller(true)]
public class WindowsPowerShell1SnapIn : PSSnapIn
{
public override string Name
{
get { return "MyFirstSnapIn"; }
}
public override string Vendor
{
get { return "Reeta"; }
}
public override string VendorResource
{
get { return "WindowsPowerShell1,"; }
}
public override string Description
{
get { return "Registers the CmdLets and Providers in this assembly"; }
}
public override string DescriptionResource
{
get { return "WindowsPowerShell1,Registers the CmdLets and Providers in this assembly"; }
}
}
3) Now add a new cmdlet class.
4) In this cmdlet we simply add a value to a parameter and output it.
[Cmdlet(VerbsCommon.Get, "Hello", SupportsShouldProcess = true)]
In this attribute “Get” is our verb and “Proc” is subject. Different verbs can be used. Read http://msdn.microsoft.com/en-us/library/ms714428(VS.85).aspx for more information.
5) To add parameter write :
[Parameter(Position = 0,
Mandatory = true,
ValueFromPipelineByPropertyName = true,
HelpMessage = "Enter a name")]
[ValidateNotNullOrEmpty]
public string Name
{
get;
set;
}
To validate a parameter we can add different attribute on a parameter. We can add help message for the parameter By “HelpMessage”. We can indicate that a parameter is mandatory by adding “Mandatory= true”. We can add position for a parameter.
6) Now add a code to write the parameter value in ProcessRecord(). When this command runs it execute code written in Processrecord().
WriteObject will write values on the commandlet.
7) For running the power shell we first need to install the dll.
On visual studio command prompt use - installUtil yourAssemblyPath.dll
8) Now add the SnapIn in the power shell using command:
Add-PSSnapin MyFirstSnapIn
9) Now get the registered SnapIn list by command :
Get-PSSnapIn –registered
10) Now run the command using:
Get-Hello –Name Reeta
It will output – Hello Reeta
Since we had written position in the Parameter attribute we don’t need to specify –Name. if we write- Get-Hello Reeta, it gives same output.
If we don’t provide any parameter then it says that it is mandatory.
These are just simple steps for creating a command in powerShell. I get help from this blog http://blogs.msdn.com/b/daiken/archive/2007/02/07/creating-a-windows-powerShell-cmdlet-using-the-visual-studio-windows-powerShell-templates.aspx