Step 1: Create a new website. In Default.aspx page put this code:
<table>
<tr>
<td>
<img height="30" alt="" src="BuildCaptcha.aspx" width="80" />
</td>
</tr>
<tr>
<td>
<asp:TextBox runat="Server" ID="txtCaptcha"/>
</td>
<td>
<asp:Button runat="Server" ID="btnSubmit" OnClick="btnSubmit_Click" Text="Submit" />
</td>
</tr>
</table>
Step 2: In Default.aspx.cs page write this code for Submit button click:
protected void btnSubmit_Click(object sender, EventArgs e)
{
if (Page.IsValid && (txtCaptcha.Text.ToString() == Session["RandomStr"].ToString()))
{
Response.Write("Code verification Successful.");
txtCaptcha.Text = string.Empty;
}
else
{
Response.Write("Please enter code correctly.");
}
}
Step 3: Now add a new Page named BuildCaptcha.aspx.
On this page we choose random numbers and create a bitmap image from them. To do this write this code on the page load:
protected void Page_Load(object sender, EventArgs e)
{
//That is to create the random # and add it to our string
Bitmap objBMP = new Bitmap(60, 20);
Graphics objGraphics = Graphics.FromImage(objBMP);
objGraphics.Clear(Color.YellowGreen);
objGraphics.TextRenderingHint = TextRenderingHint.AntiAlias;
//' Configure font to use for text
Font objFont = new Font("Arial", 8, FontStyle.Italic);
string randomStr = "";
char[] myArray = new char[5];
int x;
Random autoRand = new Random();
for (x = 0; x < 5; x++)
{
myArray[x] = System.Convert.ToChar(autoRand.Next(65, 90));
randomStr += (myArray[x].ToString());
}
//This is to add the string to session, to be compared later
Session.Add("RandomStr", randomStr);
//' Write out the text
objGraphics.DrawString(randomStr, objFont, Brushes.Blue, 3, 3);
//' Set the content type and return the image
Response.ContentType = "image/GIF";
objBMP.Save(Response.OutputStream, ImageFormat.Gif);
objFont.Dispose();
objGraphics.Dispose();
objBMP.Dispose();
}
Now you are done. When you run website it looks like this:
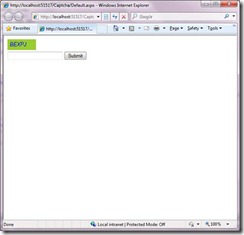
<table>
<tr>
<td>
<img height="30" alt="" src="BuildCaptcha.aspx" width="80" />
</td>
</tr>
<tr>
<td>
<asp:TextBox runat="Server" ID="txtCaptcha"/>
</td>
<td>
<asp:Button runat="Server" ID="btnSubmit" OnClick="btnSubmit_Click" Text="Submit" />
</td>
</tr>
</table>
Step 2: In Default.aspx.cs page write this code for Submit button click:
protected void btnSubmit_Click(object sender, EventArgs e)
{
if (Page.IsValid && (txtCaptcha.Text.ToString() == Session["RandomStr"].ToString()))
{
Response.Write("Code verification Successful.");
txtCaptcha.Text = string.Empty;
}
else
{
Response.Write("Please enter code correctly.");
}
}
Step 3: Now add a new Page named BuildCaptcha.aspx.
On this page we choose random numbers and create a bitmap image from them. To do this write this code on the page load:
protected void Page_Load(object sender, EventArgs e)
{
//That is to create the random # and add it to our string
Bitmap objBMP = new Bitmap(60, 20);
Graphics objGraphics = Graphics.FromImage(objBMP);
objGraphics.Clear(Color.YellowGreen);
objGraphics.TextRenderingHint = TextRenderingHint.AntiAlias;
//' Configure font to use for text
Font objFont = new Font("Arial", 8, FontStyle.Italic);
string randomStr = "";
char[] myArray = new char[5];
int x;
Random autoRand = new Random();
for (x = 0; x < 5; x++)
{
myArray[x] = System.Convert.ToChar(autoRand.Next(65, 90));
randomStr += (myArray[x].ToString());
}
//This is to add the string to session, to be compared later
Session.Add("RandomStr", randomStr);
//' Write out the text
objGraphics.DrawString(randomStr, objFont, Brushes.Blue, 3, 3);
//' Set the content type and return the image
Response.ContentType = "image/GIF";
objBMP.Save(Response.OutputStream, ImageFormat.Gif);
objFont.Dispose();
objGraphics.Dispose();
objBMP.Dispose();
}
Now you are done. When you run website it looks like this:
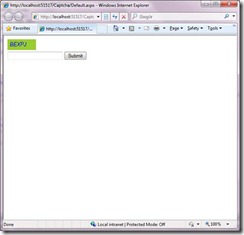